mirror of
https://github.com/sjwhitworth/golearn.git
synced 2025-04-25 13:48:49 +08:00
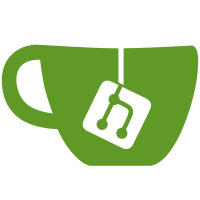
* Only numeric and categorical ARFF attributes are currently supported. * Only the dense version of the ARFF format is supported. * Compressed format is .tar.gz file which should allow extensibility. * Attributes stored using JSON representations. * Also offers smarter estimation of the precision of numeric Attributes. * Also adds support for writing instances to CSV
39 lines
976 B
Go
39 lines
976 B
Go
package linear_models
|
|
|
|
import (
|
|
"github.com/sjwhitworth/golearn/base"
|
|
. "github.com/smartystreets/goconvey/convey"
|
|
"testing"
|
|
)
|
|
|
|
func TestLogisticRegression(t *testing.T) {
|
|
Convey("Given labels, a classifier and data", t, func() {
|
|
// Load data
|
|
X, err := base.ParseCSVToInstances("train.csv", false)
|
|
So(err, ShouldEqual, nil)
|
|
Y, err := base.ParseCSVToInstances("test.csv", false)
|
|
So(err, ShouldEqual, nil)
|
|
|
|
// Setup the problem
|
|
lr, err := NewLogisticRegression("l2", 1.0, 1e-6)
|
|
So(err, ShouldBeNil)
|
|
|
|
lr.Fit(X)
|
|
|
|
Convey("When predicting the label of first vector", func() {
|
|
Z, err := lr.Predict(Y)
|
|
So(err, ShouldEqual, nil)
|
|
Convey("The result should be 1", func() {
|
|
So(Z.RowString(0), ShouldEqual, "1.0")
|
|
})
|
|
})
|
|
Convey("When predicting the label of second vector", func() {
|
|
Z, err := lr.Predict(Y)
|
|
So(err, ShouldEqual, nil)
|
|
Convey("The result should be -1", func() {
|
|
So(Z.RowString(1), ShouldEqual, "-1.0")
|
|
})
|
|
})
|
|
})
|
|
}
|