mirror of
https://github.com/sjwhitworth/golearn.git
synced 2025-04-25 13:48:49 +08:00
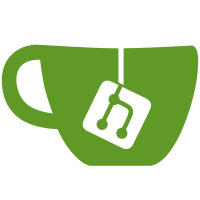
* Only numeric and categorical ARFF attributes are currently supported. * Only the dense version of the ARFF format is supported. * Compressed format is .tar.gz file which should allow extensibility. * Attributes stored using JSON representations. * Also offers smarter estimation of the precision of numeric Attributes. * Also adds support for writing instances to CSV
73 lines
2.2 KiB
Go
73 lines
2.2 KiB
Go
package base
|
|
|
|
import (
|
|
. "github.com/smartystreets/goconvey/convey"
|
|
"testing"
|
|
)
|
|
|
|
func TestLazySortDesc(t *testing.T) {
|
|
Convey("Given data that's not already sorted descending", t, func() {
|
|
unsorted, err := ParseCSVToInstances("../examples/datasets/iris_headers.csv", true)
|
|
So(err, ShouldBeNil)
|
|
|
|
as1 := ResolveAllAttributes(unsorted)
|
|
So(isSortedDesc(unsorted, as1[0]), ShouldBeFalse)
|
|
|
|
Convey("Given reference data that's alredy sorted descending", func() {
|
|
sortedDescending, err := ParseCSVToInstances("../examples/datasets/iris_sorted_desc.csv", true)
|
|
So(err, ShouldBeNil)
|
|
|
|
as2 := ResolveAllAttributes(sortedDescending)
|
|
So(isSortedDesc(sortedDescending, as2[0]), ShouldBeTrue)
|
|
|
|
Convey("LazySorting Descending", func() {
|
|
result, err := LazySort(unsorted, Descending, as1[0:len(as1)-1])
|
|
So(err, ShouldBeNil)
|
|
|
|
Convey("Result should be sorted descending", func() {
|
|
So(isSortedDesc(result, as1[0]), ShouldBeTrue)
|
|
})
|
|
|
|
Convey("Result should match the reference", func() {
|
|
So(InstancesAreEqual(sortedDescending, result), ShouldBeTrue)
|
|
})
|
|
})
|
|
})
|
|
})
|
|
}
|
|
|
|
func TestLazySortAsc(t *testing.T) {
|
|
Convey("Given data that's not already sorted ascending", t, func() {
|
|
unsorted, err := ParseCSVToInstances("../examples/datasets/iris_headers.csv", true)
|
|
So(err, ShouldBeNil)
|
|
|
|
as1 := ResolveAllAttributes(unsorted)
|
|
So(isSortedAsc(unsorted, as1[0]), ShouldBeFalse)
|
|
|
|
Convey("Given reference data that's alredy sorted ascending", func() {
|
|
sortedAscending, err := ParseCSVToInstances("../examples/datasets/iris_sorted_asc.csv", true)
|
|
So(err, ShouldBeNil)
|
|
|
|
as2 := ResolveAllAttributes(sortedAscending)
|
|
So(isSortedAsc(sortedAscending, as2[0]), ShouldBeTrue)
|
|
|
|
Convey("LazySorting Ascending", func() {
|
|
result, err := LazySort(unsorted, Ascending, as1[0:len(as1)-1])
|
|
So(err, ShouldBeNil)
|
|
|
|
Convey("Result should be sorted descending", func() {
|
|
So(isSortedAsc(result, as1[0]), ShouldBeTrue)
|
|
})
|
|
|
|
Convey("Result should match the reference", func() {
|
|
So(InstancesAreEqual(sortedAscending, result), ShouldBeTrue)
|
|
})
|
|
|
|
Convey("First element of Result should equal known value", func() {
|
|
So(result.RowString(0), ShouldEqual, "4.3 3.0 1.1 0.1 Iris-setosa")
|
|
})
|
|
})
|
|
})
|
|
})
|
|
}
|