mirror of
https://github.com/unidoc/unipdf.git
synced 2025-05-02 22:17:06 +08:00
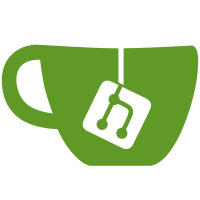
* Add render package * Add text state * Add more text operators * Remove unnecessary files * Add text font * Add custom text render method * Improve text rendering method * Rename text state methods * Refactor and document context interface * Refact text begin/end operators * Fix graphics state transformations * Keep original font when doing font substitution * Take page cropbox into account * Revert to substitution font if original font measurement is 0 * Add font substitution package * Implement addition transform.Point methods * Use transform.Point in the image context package * Remove unneeded functionality from the render image package * Fix golint notices in the image rendering package * Fix go vet notices in the render package * Fix golint notices in the top-level render package * Improve render context package documentation * Document context text state struct. * Document context text font struct. * Minor logging improvements * Add license disclaimer to the render package files * Avoid using package aliases where possible * Change style of section comments * Adapt render package import style to follow the developer guide * Improve documentation for the internal matrix implementation * Update render package dependency versions * Apply crop box post render * Account for offseted media boxes * Improve metrics of rendered characters * Fix text matrix translation * Change priority of fonts used for measuring rendered characters * Skip invalid m and l operators on image rendering * Small fix for v operator * Fix rendered characters spacing issues * Refactor naming of internal render packages
69 lines
1.4 KiB
Go
69 lines
1.4 KiB
Go
/*
|
|
* This file is subject to the terms and conditions defined in
|
|
* file 'LICENSE.md', which is part of this source code package.
|
|
*/
|
|
|
|
package imagerender
|
|
|
|
import (
|
|
"math"
|
|
|
|
"github.com/unidoc/unipdf/v3/internal/transform"
|
|
)
|
|
|
|
func quadratic(x0, y0, x1, y1, x2, y2, t float64) (x, y float64) {
|
|
u := 1 - t
|
|
a := u * u
|
|
b := 2 * u * t
|
|
c := t * t
|
|
x = a*x0 + b*x1 + c*x2
|
|
y = a*y0 + b*y1 + c*y2
|
|
return
|
|
}
|
|
|
|
func quadraticBezier(x0, y0, x1, y1, x2, y2 float64) []transform.Point {
|
|
l := (math.Hypot(x1-x0, y1-y0) +
|
|
math.Hypot(x2-x1, y2-y1))
|
|
n := int(l + 0.5)
|
|
if n < 4 {
|
|
n = 4
|
|
}
|
|
d := float64(n) - 1
|
|
result := make([]transform.Point, n)
|
|
for i := 0; i < n; i++ {
|
|
t := float64(i) / d
|
|
x, y := quadratic(x0, y0, x1, y1, x2, y2, t)
|
|
result[i] = transform.NewPoint(x, y)
|
|
}
|
|
return result
|
|
}
|
|
|
|
func cubic(x0, y0, x1, y1, x2, y2, x3, y3, t float64) (x, y float64) {
|
|
u := 1 - t
|
|
a := u * u * u
|
|
b := 3 * u * u * t
|
|
c := 3 * u * t * t
|
|
d := t * t * t
|
|
x = a*x0 + b*x1 + c*x2 + d*x3
|
|
y = a*y0 + b*y1 + c*y2 + d*y3
|
|
return
|
|
}
|
|
|
|
func cubicBezier(x0, y0, x1, y1, x2, y2, x3, y3 float64) []transform.Point {
|
|
l := (math.Hypot(x1-x0, y1-y0) +
|
|
math.Hypot(x2-x1, y2-y1) +
|
|
math.Hypot(x3-x2, y3-y2))
|
|
n := int(l + 0.5)
|
|
if n < 4 {
|
|
n = 4
|
|
}
|
|
d := float64(n) - 1
|
|
result := make([]transform.Point, n)
|
|
for i := 0; i < n; i++ {
|
|
t := float64(i) / d
|
|
x, y := cubic(x0, y0, x1, y1, x2, y2, x3, y3, t)
|
|
result[i] = transform.NewPoint(x, y)
|
|
}
|
|
return result
|
|
}
|