mirror of
https://github.com/unidoc/unipdf.git
synced 2025-05-02 22:17:06 +08:00
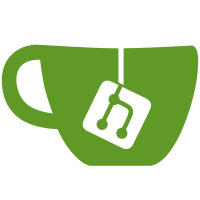
* Add render package * Add text state * Add more text operators * Remove unnecessary files * Add text font * Add custom text render method * Improve text rendering method * Rename text state methods * Refactor and document context interface * Refact text begin/end operators * Fix graphics state transformations * Keep original font when doing font substitution * Take page cropbox into account * Revert to substitution font if original font measurement is 0 * Add font substitution package * Implement addition transform.Point methods * Use transform.Point in the image context package * Remove unneeded functionality from the render image package * Fix golint notices in the image rendering package * Fix go vet notices in the render package * Fix golint notices in the top-level render package * Improve render context package documentation * Document context text state struct. * Document context text font struct. * Minor logging improvements * Add license disclaimer to the render package files * Avoid using package aliases where possible * Change style of section comments * Adapt render package import style to follow the developer guide * Improve documentation for the internal matrix implementation * Update render package dependency versions * Apply crop box post render * Account for offseted media boxes * Improve metrics of rendered characters * Fix text matrix translation * Change priority of fonts used for measuring rendered characters * Skip invalid m and l operators on image rendering * Small fix for v operator * Fix rendered characters spacing issues * Refactor naming of internal render packages
74 lines
1.3 KiB
Go
74 lines
1.3 KiB
Go
/*
|
|
* This file is subject to the terms and conditions defined in
|
|
* file 'LICENSE.md', which is part of this source code package.
|
|
*/
|
|
|
|
package imagerender
|
|
|
|
import (
|
|
"fmt"
|
|
"image"
|
|
"image/draw"
|
|
"math"
|
|
"strings"
|
|
|
|
"golang.org/x/image/math/fixed"
|
|
|
|
"github.com/unidoc/unipdf/v3/internal/transform"
|
|
)
|
|
|
|
func degreesToRadians(degrees float64) float64 {
|
|
return degrees * math.Pi / 180
|
|
}
|
|
|
|
func imageToRGBA(src image.Image) *image.RGBA {
|
|
bounds := src.Bounds()
|
|
dst := image.NewRGBA(bounds)
|
|
draw.Draw(dst, bounds, src, bounds.Min, draw.Src)
|
|
return dst
|
|
}
|
|
|
|
func parseHexColor(x string) (r, g, b, a int) {
|
|
x = strings.TrimPrefix(x, "#")
|
|
a = 255
|
|
if len(x) == 3 {
|
|
format := "%1x%1x%1x"
|
|
fmt.Sscanf(x, format, &r, &g, &b)
|
|
r |= r << 4
|
|
g |= g << 4
|
|
b |= b << 4
|
|
}
|
|
if len(x) == 6 {
|
|
format := "%02x%02x%02x"
|
|
fmt.Sscanf(x, format, &r, &g, &b)
|
|
}
|
|
if len(x) == 8 {
|
|
format := "%02x%02x%02x%02x"
|
|
fmt.Sscanf(x, format, &r, &g, &b, &a)
|
|
}
|
|
return
|
|
}
|
|
|
|
func fixedPoint(p transform.Point) fixed.Point26_6 {
|
|
return fixed.Point26_6{
|
|
X: fix(p.X),
|
|
Y: fix(p.Y),
|
|
}
|
|
}
|
|
|
|
func fix(x float64) fixed.Int26_6 {
|
|
return fixed.Int26_6(x * 64)
|
|
}
|
|
|
|
func unfix(x fixed.Int26_6) float64 {
|
|
const shift, mask = 6, 1<<6 - 1
|
|
if x >= 0 {
|
|
return float64(x>>shift) + float64(x&mask)/64
|
|
}
|
|
x = -x
|
|
if x >= 0 {
|
|
return -(float64(x>>shift) + float64(x&mask)/64)
|
|
}
|
|
return 0
|
|
}
|