mirror of
https://github.com/unidoc/unipdf.git
synced 2025-05-05 19:30:30 +08:00
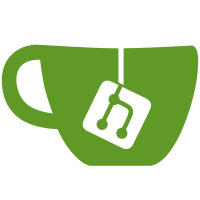
* Prepared skeleton and basic component implementations for the jbig2 encoding. * Added Bitset. Implemented Bitmap. * Decoder with old Arithmetic Decoder * Partly working arithmetic * Working arithmetic decoder. * MMR patched. * rebuild to apache. * Working generic * Working generic * Decoded full document * Update Jenkinsfile go version [master] (#398) * Update Jenkinsfile go version * Decoded AnnexH document * Minor issues fixed. * Update README.md * Fixed generic region errors. Added benchmark. Added bitmap unpadder. Added Bitmap toImage method. * Fixed endofpage error * Added integration test. * Decoded all test files without errors. Implemented JBIG2Global. * Merged with v3 version * Fixed the EOF in the globals issue * Fixed the JBIG2 ChocolateData Decode * JBIG2 Added license information * Minor fix in jbig2 encoding. * Applied the logging convention * Cleaned unnecessary imports * Go modules clear unused imports * checked out the README.md * Moved trace to Debug. Fixed the build integrate tag in the document_decode_test.go * Initial encoder skeleton * Applied UniPDF Developer Guide. Fixed lint issues. * Cleared documentation, fixed style issues. * Added jbig2 doc.go files. Applied unipdf guide style. * Minor code style changes. * Minor naming and style issues fixes. * Minor naming changes. Style issues fixed. * Review r11 fixes. * Added JBIG2 Encoder skeleton. * Moved Document and Page to jbig2/document package. Created decoder package responsible for decoding jbig2 stream. * Implemented raster functions. * Added raster uni low test funcitons. * Added raster low test functions * untracked files on jbig2-encoder: c869089 Added raster low test functions * index on jbig2-encoder: c869089 Added raster low test functions * Added morph files. * implemented jbig2 encoder basics * JBIG2 Encoder - Generic method * Added jbig2 image encode ttests, black/white image tests * cleaned and tested jbig2 package * unfinished jbig2 classified encoder * jbig2 minor style changes * minor jbig2 encoder changes * prepared JBIG2 Encoder * Style and lint fixes * Minor changes and lints * Fixed shift unsinged value build errors * Minor naming change * Added jbig2 encode, image gondels. Fixed jbig2 decode bug. * Provided jbig2 core.DecodeGlobals function. * Fixed JBIG2Encoder `r6` revision issues. * Removed public JBIG2Encoder document. * Minor style changes * added NewJBIG2Encoder function. * fixed JBIG2Encoder 'r9' revision issues. * Cleared 'r9' commented code. * Updated ACKNOWLEDGEMENETS. Fixed JBIG2Encoder 'r10' revision issues. Co-authored-by: Gunnsteinn Hall <gunnsteinn.hall@gmail.com>
82 lines
1.9 KiB
Go
82 lines
1.9 KiB
Go
/*
|
|
* This file is subject to the terms and conditions defined in
|
|
* file 'LICENSE.md', which is part of this source code package.
|
|
*/
|
|
|
|
package classer
|
|
|
|
import (
|
|
"github.com/unidoc/unipdf/v3/common"
|
|
|
|
"github.com/unidoc/unipdf/v3/internal/jbig2/bitmap"
|
|
)
|
|
|
|
// similarTemplatesFinder stores the state of a state machine which fetches similar sized templates.
|
|
type similarTemplatesFinder struct {
|
|
Classer *Classer
|
|
// Desired width
|
|
Width int
|
|
// Desired height
|
|
Height int
|
|
// Index into two_by_two step array
|
|
Index int
|
|
// Current number array
|
|
CurrentNumbers []int
|
|
// Current element of the array
|
|
N int
|
|
}
|
|
|
|
// initSimilarTemplatesFinder initializes the templatesState context.
|
|
func initSimilarTemplatesFinder(c *Classer, bms *bitmap.Bitmap) *similarTemplatesFinder {
|
|
return &similarTemplatesFinder{
|
|
Width: bms.Width,
|
|
Height: bms.Height,
|
|
Classer: c,
|
|
}
|
|
}
|
|
|
|
// Next finds next template state.
|
|
func (f *similarTemplatesFinder) Next() int {
|
|
var (
|
|
desireDH, desireDW, size, templ int
|
|
ok bool
|
|
bmT *bitmap.Bitmap
|
|
err error
|
|
)
|
|
|
|
for {
|
|
if f.Index >= 25 {
|
|
return -1
|
|
}
|
|
desireDW = f.Width + TwoByTwoWalk[2*f.Index]
|
|
desireDH = f.Height + TwoByTwoWalk[2*f.Index+1]
|
|
if desireDH < 1 || desireDW < 1 {
|
|
f.Index++
|
|
continue
|
|
}
|
|
|
|
if len(f.CurrentNumbers) == 0 {
|
|
f.CurrentNumbers, ok = f.Classer.TemplatesSize.GetSlice(uint64(desireDW) * uint64(desireDH))
|
|
if !ok {
|
|
f.Index++
|
|
continue
|
|
}
|
|
f.N = 0
|
|
}
|
|
size = len(f.CurrentNumbers)
|
|
for ; f.N < size; f.N++ {
|
|
templ = f.CurrentNumbers[f.N]
|
|
bmT, err = f.Classer.DilatedTemplates.GetBitmap(templ)
|
|
if err != nil {
|
|
common.Log.Debug("FindNextTemplate: template not found: ")
|
|
return 0
|
|
}
|
|
if bmT.Width-2*JbAddedPixels == desireDW && bmT.Height-2*JbAddedPixels == desireDH {
|
|
return templ
|
|
}
|
|
}
|
|
f.Index++
|
|
f.CurrentNumbers = nil
|
|
}
|
|
}
|