mirror of
https://github.com/gdamore/tcell.git
synced 2025-04-24 13:48:51 +08:00
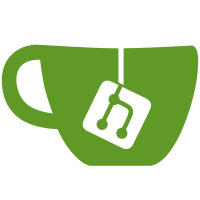
The API for NewSimulationScreen() was changed, which turned out to break 3rd party libraries and applications. (We didn't forgot that this is part of the public API for tcell.)
110 lines
2.1 KiB
Go
110 lines
2.1 KiB
Go
// Copyright 2018 The TCell Authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use file except in compliance with the License.
|
|
// You may obtain a copy of the license at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package tcell
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func eventLoop(s Screen, evch chan Event) {
|
|
for {
|
|
ev := s.PollEvent()
|
|
if ev == nil {
|
|
close(evch)
|
|
return
|
|
}
|
|
select {
|
|
case evch <- ev:
|
|
case <-time.After(time.Second):
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestMouseEvents(t *testing.T) {
|
|
|
|
s := mkTestScreen(t, "")
|
|
defer s.Fini()
|
|
|
|
s.EnableMouse()
|
|
s.InjectMouse(4, 9, Button1, ModCtrl)
|
|
evch := make(chan Event)
|
|
em := &EventMouse{}
|
|
done := false
|
|
go eventLoop(s, evch)
|
|
|
|
for !done {
|
|
select {
|
|
case ev := <-evch:
|
|
if evm, ok := ev.(*EventMouse); ok {
|
|
em = evm
|
|
done = true
|
|
}
|
|
continue
|
|
case <-time.After(time.Second):
|
|
done = true
|
|
}
|
|
}
|
|
|
|
if x, y := em.Position(); x != 4 || y != 9 {
|
|
t.Errorf("Mouse position wrong (%v, %v)", x, y)
|
|
}
|
|
if em.Buttons() != Button1 {
|
|
t.Errorf("Should be Button1")
|
|
}
|
|
if em.Modifiers() != ModCtrl {
|
|
t.Errorf("Modifiers should be control")
|
|
}
|
|
}
|
|
|
|
func TestChannelMouseEvents(t *testing.T) {
|
|
|
|
s := mkTestScreen(t, "")
|
|
defer s.Fini()
|
|
|
|
s.EnableMouse()
|
|
s.InjectMouse(4, 9, Button1, ModCtrl)
|
|
evch := make(chan Event)
|
|
quit := make(chan struct{})
|
|
em := new(EventMouse)
|
|
go s.ChannelEvents(evch, quit)
|
|
|
|
loop:
|
|
for {
|
|
select {
|
|
case ev := <-evch:
|
|
if evm, ok := ev.(*EventMouse); ok {
|
|
em = evm
|
|
close(quit)
|
|
break loop
|
|
}
|
|
continue
|
|
case <-time.After(time.Second):
|
|
close(quit)
|
|
break loop
|
|
}
|
|
}
|
|
|
|
if x, y := em.Position(); x != 4 || y != 9 {
|
|
t.Errorf("Mouse position wrong (%v, %v)", x, y)
|
|
}
|
|
if em.Buttons() != Button1 {
|
|
t.Errorf("Should be Button1")
|
|
}
|
|
if em.Modifiers() != ModCtrl {
|
|
t.Errorf("Modifiers should be control")
|
|
}
|
|
}
|