mirror of
https://github.com/shirou/gopsutil.git
synced 2025-04-28 13:48:49 +08:00
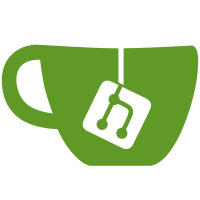
The order of init function execution is dependant on the order that the source files are passed to the compiler. This causes issues when building under other build systems, such as bazel or buck, as they are not guarenteed to maintain the same file order as the default go tool.
61 lines
1.6 KiB
Go
61 lines
1.6 KiB
Go
package disk
|
|
|
|
import (
|
|
"encoding/json"
|
|
|
|
"github.com/shirou/gopsutil/internal/common"
|
|
)
|
|
|
|
var invoke common.Invoker = common.Invoke{}
|
|
|
|
type UsageStat struct {
|
|
Path string `json:"path"`
|
|
Fstype string `json:"fstype"`
|
|
Total uint64 `json:"total"`
|
|
Free uint64 `json:"free"`
|
|
Used uint64 `json:"used"`
|
|
UsedPercent float64 `json:"usedPercent"`
|
|
InodesTotal uint64 `json:"inodesTotal"`
|
|
InodesUsed uint64 `json:"inodesUsed"`
|
|
InodesFree uint64 `json:"inodesFree"`
|
|
InodesUsedPercent float64 `json:"inodesUsedPercent"`
|
|
}
|
|
|
|
type PartitionStat struct {
|
|
Device string `json:"device"`
|
|
Mountpoint string `json:"mountpoint"`
|
|
Fstype string `json:"fstype"`
|
|
Opts string `json:"opts"`
|
|
}
|
|
|
|
type IOCountersStat struct {
|
|
ReadCount uint64 `json:"readCount"`
|
|
MergedReadCount uint64 `json:"mergedReadCount"`
|
|
WriteCount uint64 `json:"writeCount"`
|
|
MergedWriteCount uint64 `json:"mergedWriteCount"`
|
|
ReadBytes uint64 `json:"readBytes"`
|
|
WriteBytes uint64 `json:"writeBytes"`
|
|
ReadTime uint64 `json:"readTime"`
|
|
WriteTime uint64 `json:"writeTime"`
|
|
IopsInProgress uint64 `json:"iopsInProgress"`
|
|
IoTime uint64 `json:"ioTime"`
|
|
WeightedIO uint64 `json:"weightedIO"`
|
|
Name string `json:"name"`
|
|
SerialNumber string `json:"serialNumber"`
|
|
}
|
|
|
|
func (d UsageStat) String() string {
|
|
s, _ := json.Marshal(d)
|
|
return string(s)
|
|
}
|
|
|
|
func (d PartitionStat) String() string {
|
|
s, _ := json.Marshal(d)
|
|
return string(s)
|
|
}
|
|
|
|
func (d IOCountersStat) String() string {
|
|
s, _ := json.Marshal(d)
|
|
return string(s)
|
|
}
|