mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-26 13:48:53 +08:00
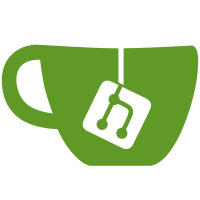
* Add list channels by thing id endpoint Add list channels by thing id endpoint to things service. Add pagination format and logic. Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add fetch channels by thing endpoint Add fetch channels by thing endpoint with pagination. Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Update list endpoints to contain pagination Update list endpoints to contain pagination metadata. Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add tests for two new endpoints Add tests for two new endpoints and update existing ones. Also, remove connected field from channel response. Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Fix tests for SDK Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add SDK methods for new endpoints Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Update swagger docs for things service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add error handling to http tests Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Fix response body in http tests Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Remove unused responses from things service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add test cases to things postgres tests Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add test cases for event sourcing Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com>
84 lines
2.1 KiB
Go
84 lines
2.1 KiB
Go
//
|
|
// Copyright (c) 2018
|
|
// Mainflux
|
|
//
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
//
|
|
|
|
package things
|
|
|
|
import "strings"
|
|
|
|
// Thing represents a Mainflux thing. Each thing is owned by one user, and
|
|
// it is assigned with the unique identifier and (temporary) access key.
|
|
type Thing struct {
|
|
ID string
|
|
Owner string
|
|
Type string
|
|
Name string
|
|
Key string
|
|
Metadata string
|
|
}
|
|
|
|
// ThingsPage contains page related metadata as well as list of things that
|
|
// belong to this page.
|
|
type ThingsPage struct {
|
|
PageMetadata
|
|
Things []Thing
|
|
}
|
|
|
|
var thingTypes = map[string]bool{
|
|
"app": true,
|
|
"device": true,
|
|
}
|
|
|
|
// Validate returns an error if thing representation is invalid.
|
|
func (c *Thing) Validate() error {
|
|
if c.Type = strings.ToLower(c.Type); !thingTypes[c.Type] {
|
|
return ErrMalformedEntity
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ThingRepository specifies a thing persistence API.
|
|
type ThingRepository interface {
|
|
// Save persists the thing. Successful operation is indicated by non-nil
|
|
// error response.
|
|
Save(Thing) (string, error)
|
|
|
|
// Update performs an update to the existing thing. A non-nil error is
|
|
// returned to indicate operation failure.
|
|
Update(Thing) error
|
|
|
|
// RetrieveByID retrieves the thing having the provided identifier, that is owned
|
|
// by the specified user.
|
|
RetrieveByID(string, string) (Thing, error)
|
|
|
|
// RetrieveByKey returns thing ID for given thing key.
|
|
RetrieveByKey(string) (string, error)
|
|
|
|
// RetrieveAll retrieves the subset of things owned by the specified user.
|
|
RetrieveAll(string, uint64, uint64) ThingsPage
|
|
|
|
// RetrieveByChannel retrieves the subset of things owned by the specified
|
|
// user and connected to specified channel.
|
|
RetrieveByChannel(string, string, uint64, uint64) ThingsPage
|
|
|
|
// Remove removes the thing having the provided identifier, that is owned
|
|
// by the specified user.
|
|
Remove(string, string) error
|
|
}
|
|
|
|
// ThingCache contains thing caching interface.
|
|
type ThingCache interface {
|
|
// Save stores pair thing key, thing id.
|
|
Save(string, string) error
|
|
|
|
// ID returns thing ID for given key.
|
|
ID(string) (string, error)
|
|
|
|
// Removes thing from cache.
|
|
Remove(string) error
|
|
}
|