mirror of
https://github.com/mainflux/mainflux.git
synced 2025-05-14 19:29:11 +08:00
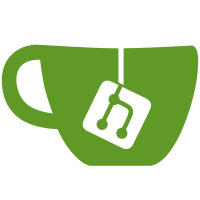
* NOISSUE- Add OPC-UA adapter Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * NOISSUE - Add opc-adapter PoC, docker and vendor Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Convert OPC messages to SenML Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add gopcua package Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * lora-adapter typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add OPC Reader Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Typo fix Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Typo fix Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Update copyright headers Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix reviews Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix reviews Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add opc config Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add all opc envars in the config Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Config typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add route map Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Use opcua package instead of opc Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix OPCUA typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Rm MQTT sub Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Move interefaces to root Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix revieews and typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Update Gopkg.toml Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add all envars into .env Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com>
51 lines
1.8 KiB
Go
51 lines
1.8 KiB
Go
// Copyright 2018-2019 opcua authors. All rights reserved.
|
||
// Use of this source code is governed by a MIT-style license that can be
|
||
// found in the LICENSE file.
|
||
|
||
package uapolicy
|
||
|
||
import (
|
||
"crypto/rsa"
|
||
)
|
||
|
||
/*
|
||
"SecurityPolicy – None" Profile
|
||
http://opcfoundation.org/UA/SecurityPolicy#None
|
||
|
||
Security None CreateSession ActivateSession
|
||
When SecurityPolicy=None, the CreateSession and ActivateSession service allow for a NULL/empty signature and do not require Application Certificates or a Nonce.
|
||
(OPT) Security None CreateSession ActivateSession 1.0
|
||
The Client can connect to Servers that require a certificate being passed on Session establishment.
|
||
The Client in this case will first try without a certificate and if this fails present a certificate.
|
||
SymmetricSignatureAlgorithm_None This algorithm does not apply.
|
||
SymmetricEncryptionAlgorithm_None This algorithm does not apply.
|
||
AsymmetricSignatureAlgorithm_None This algorithm does not apply.
|
||
AsymmetricEncryptionAlgorithm_None This algorithm does not apply.
|
||
KeyDerivationAlgorithm_None This algorithm does not apply.
|
||
SecurityPolicy_None_Limits DerivedSignatureKeyLength: 0
|
||
|
||
*/
|
||
func newNoneAsymmetric(*rsa.PrivateKey, *rsa.PublicKey) (*EncryptionAlgorithm, error) {
|
||
return &EncryptionAlgorithm{
|
||
blockSize: NoneBlockSize,
|
||
plainttextBlockSize: NoneBlockSize - NoneMinPadding,
|
||
encrypt: &None{},
|
||
decrypt: &None{},
|
||
signature: &None{},
|
||
verifySignature: &None{},
|
||
signatureLength: 0,
|
||
}, nil
|
||
}
|
||
|
||
func newNoneSymmetric([]byte, []byte) (*EncryptionAlgorithm, error) {
|
||
return &EncryptionAlgorithm{
|
||
blockSize: NoneBlockSize,
|
||
plainttextBlockSize: NoneBlockSize - NoneMinPadding,
|
||
encrypt: &None{},
|
||
decrypt: &None{},
|
||
signature: &None{},
|
||
verifySignature: &None{},
|
||
signatureLength: 0,
|
||
}, nil
|
||
}
|