mirror of
https://github.com/mainflux/mainflux.git
synced 2025-05-14 19:29:11 +08:00
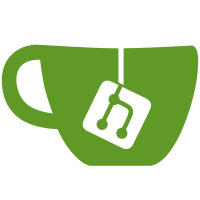
* NOISSUE- Add OPC-UA adapter Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * NOISSUE - Add opc-adapter PoC, docker and vendor Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Convert OPC messages to SenML Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add gopcua package Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * lora-adapter typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add OPC Reader Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Typo fix Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Typo fix Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Update copyright headers Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix reviews Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix reviews Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add opc config Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add all opc envars in the config Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Config typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add route map Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Use opcua package instead of opc Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix OPCUA typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Rm MQTT sub Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Move interefaces to root Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix revieews and typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Update Gopkg.toml Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add all envars into .env Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com>
114 lines
2.1 KiB
Go
114 lines
2.1 KiB
Go
package uapolicy
|
|
|
|
import (
|
|
"crypto"
|
|
"crypto/rand"
|
|
"crypto/rsa"
|
|
|
|
// Force compilation of required hashing algorithms, although we don't directly use the packages
|
|
_ "crypto/sha1"
|
|
_ "crypto/sha256"
|
|
|
|
"github.com/gopcua/opcua/ua"
|
|
)
|
|
|
|
const PKCS1v15MinPadding = 11
|
|
|
|
type PKCS1v15 struct {
|
|
Hash crypto.Hash
|
|
PublicKey *rsa.PublicKey
|
|
PrivateKey *rsa.PrivateKey
|
|
}
|
|
|
|
func (c *PKCS1v15) Decrypt(src []byte) ([]byte, error) {
|
|
if c.PrivateKey == nil {
|
|
return nil, ua.StatusBadSecurityChecksFailed
|
|
}
|
|
|
|
rng := rand.Reader
|
|
|
|
var plaintext []byte
|
|
|
|
blockSize := c.PrivateKey.PublicKey.Size()
|
|
srcRemaining := len(src)
|
|
start := 0
|
|
|
|
for srcRemaining > 0 {
|
|
end := start + blockSize
|
|
if end > len(src) {
|
|
end = len(src)
|
|
}
|
|
|
|
p, err := rsa.DecryptPKCS1v15(rng, c.PrivateKey, src[start:end])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
plaintext = append(plaintext, p...)
|
|
start = end
|
|
srcRemaining = len(src) - start
|
|
}
|
|
|
|
return plaintext, nil
|
|
}
|
|
|
|
func (c *PKCS1v15) Encrypt(src []byte) ([]byte, error) {
|
|
if c.PublicKey == nil {
|
|
return nil, ua.StatusBadSecurityChecksFailed
|
|
}
|
|
|
|
rng := rand.Reader
|
|
|
|
var ciphertext []byte
|
|
|
|
maxBlock := c.PublicKey.Size() - PKCS1v15MinPadding
|
|
srcRemaining := len(src)
|
|
start := 0
|
|
for srcRemaining > 0 {
|
|
end := start + maxBlock
|
|
if end > len(src) {
|
|
end = len(src)
|
|
}
|
|
|
|
c, err := rsa.EncryptPKCS1v15(rng, c.PublicKey, src[start:end])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
ciphertext = append(ciphertext, c...)
|
|
start = end
|
|
srcRemaining = len(src) - start
|
|
}
|
|
|
|
return ciphertext, nil
|
|
}
|
|
|
|
func (s *PKCS1v15) Signature(msg []byte) ([]byte, error) {
|
|
if s.PrivateKey == nil {
|
|
return nil, ua.StatusBadSecurityChecksFailed
|
|
}
|
|
|
|
rng := rand.Reader
|
|
|
|
h := s.Hash.New()
|
|
if _, err := h.Write(msg); err != nil {
|
|
return nil, err
|
|
}
|
|
hashed := h.Sum(nil)
|
|
|
|
return rsa.SignPKCS1v15(rng, s.PrivateKey, s.Hash, hashed[:])
|
|
}
|
|
|
|
func (s *PKCS1v15) Verify(msg, signature []byte) error {
|
|
if s.PublicKey == nil {
|
|
return ua.StatusBadSecurityChecksFailed
|
|
}
|
|
|
|
h := s.Hash.New()
|
|
if _, err := h.Write(msg); err != nil {
|
|
return err
|
|
}
|
|
hashed := h.Sum(nil)
|
|
return rsa.VerifyPKCS1v15(s.PublicKey, s.Hash, hashed[:], signature)
|
|
}
|