mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-24 13:48:49 +08:00
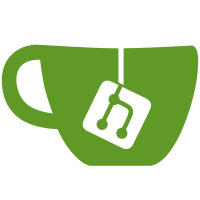
* Fix linting errors Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(linters): add ineffassign linter This commit adds the `ineffassign` linter to the project's `.golangci.yml` configuration file. The `ineffassign` linter helps identify and flag assignments to variables that are never used, helping to improve code quality and maintainability. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * Add extra linters Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(golangci): Add header check - Added goheader check to ensure all files have license headers - Added build tags for "nats" in the .golangci.yml file to include the necessary dependencies for the "nats" package during the build process. - Also, increased the maximum number of issues per linter and the maximum number of same issues reported by the linter to improve the code quality analysis. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(.golangci.yml): Add new linters Add the following new linters to the .golangci.yml configuration file: - asasalint - asciicheck - bidichk - contextcheck - decorder - dogsled - errchkjson - errname - execinquery - exportloopref - ginkgolinter - gocheckcompilerdirectives These linters will help improve code quality and catch potential issues during the code review process. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> --------- Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com>
254 lines
6.5 KiB
Go
254 lines
6.5 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package sdk
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"net/http"
|
|
"time"
|
|
|
|
"github.com/mainflux/mainflux/pkg/errors"
|
|
)
|
|
|
|
const (
|
|
policyEndpoint = "policies"
|
|
authorizeEndpoint = "authorize"
|
|
accessEndpoint = "access"
|
|
)
|
|
|
|
// Policy represents an argument struct for making a policy related function calls.
|
|
type Policy struct {
|
|
OwnerID string `json:"owner_id"`
|
|
Subject string `json:"subject"`
|
|
Object string `json:"object"`
|
|
Actions []string `json:"actions"`
|
|
External bool `json:"external,omitempty"` // This is specificially used in things service. If set to true, it means the subject is userID otherwise it is thingID.
|
|
CreatedAt time.Time `json:"created_at"`
|
|
UpdatedAt time.Time `json:"updated_at"`
|
|
}
|
|
|
|
type AccessRequest struct {
|
|
Subject string `json:"subject,omitempty"`
|
|
Object string `json:"object,omitempty"`
|
|
Action string `json:"action,omitempty"`
|
|
EntityType string `json:"entity_type,omitempty"`
|
|
}
|
|
|
|
func (sdk mfSDK) AuthorizeUser(accessReq AccessRequest, token string) (bool, errors.SDKError) {
|
|
data, err := json.Marshal(accessReq)
|
|
if err != nil {
|
|
return false, errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.usersURL, authorizeEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusOK)
|
|
if sdkerr != nil {
|
|
return false, sdkerr
|
|
}
|
|
|
|
return true, nil
|
|
}
|
|
|
|
func (sdk mfSDK) CreateUserPolicy(p Policy, token string) errors.SDKError {
|
|
data, err := json.Marshal(p)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.usersURL, policyEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusCreated)
|
|
if sdkerr != nil {
|
|
return sdkerr
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (sdk mfSDK) UpdateUserPolicy(p Policy, token string) errors.SDKError {
|
|
data, err := json.Marshal(p)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.usersURL, policyEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPut, url, token, data, nil, http.StatusNoContent)
|
|
if sdkerr != nil {
|
|
return sdkerr
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (sdk mfSDK) ListUserPolicies(pm PageMetadata, token string) (PolicyPage, errors.SDKError) {
|
|
url, err := sdk.withQueryParams(sdk.usersURL, policyEndpoint, pm)
|
|
if err != nil {
|
|
return PolicyPage{}, errors.NewSDKError(err)
|
|
}
|
|
|
|
_, body, sdkerr := sdk.processRequest(http.MethodGet, url, token, nil, nil, http.StatusOK)
|
|
if sdkerr != nil {
|
|
return PolicyPage{}, sdkerr
|
|
}
|
|
|
|
var pp PolicyPage
|
|
if err := json.Unmarshal(body, &pp); err != nil {
|
|
return PolicyPage{}, errors.NewSDKError(err)
|
|
}
|
|
|
|
return pp, nil
|
|
}
|
|
|
|
func (sdk mfSDK) DeleteUserPolicy(p Policy, token string) errors.SDKError {
|
|
url := fmt.Sprintf("%s/%s/%s/%s", sdk.usersURL, policyEndpoint, p.Subject, p.Object)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodDelete, url, token, nil, nil, http.StatusNoContent)
|
|
|
|
return sdkerr
|
|
}
|
|
|
|
func (sdk mfSDK) CreateThingPolicy(p Policy, token string) errors.SDKError {
|
|
data, err := json.Marshal(p)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.thingsURL, policyEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusCreated)
|
|
if sdkerr != nil {
|
|
return sdkerr
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (sdk mfSDK) UpdateThingPolicy(p Policy, token string) errors.SDKError {
|
|
data, err := json.Marshal(p)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.thingsURL, policyEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPut, url, token, data, nil, http.StatusNoContent)
|
|
if sdkerr != nil {
|
|
return sdkerr
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (sdk mfSDK) ListThingPolicies(pm PageMetadata, token string) (PolicyPage, errors.SDKError) {
|
|
url, err := sdk.withQueryParams(sdk.thingsURL, policyEndpoint, pm)
|
|
if err != nil {
|
|
return PolicyPage{}, errors.NewSDKError(err)
|
|
}
|
|
|
|
_, body, sdkerr := sdk.processRequest(http.MethodGet, url, token, nil, nil, http.StatusOK)
|
|
if sdkerr != nil {
|
|
return PolicyPage{}, sdkerr
|
|
}
|
|
|
|
var pp PolicyPage
|
|
if err := json.Unmarshal(body, &pp); err != nil {
|
|
return PolicyPage{}, errors.NewSDKError(err)
|
|
}
|
|
|
|
return pp, nil
|
|
}
|
|
|
|
func (sdk mfSDK) DeleteThingPolicy(p Policy, token string) errors.SDKError {
|
|
url := fmt.Sprintf("%s/%s/%s/%s", sdk.thingsURL, policyEndpoint, p.Subject, p.Object)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodDelete, url, token, nil, nil, http.StatusNoContent)
|
|
|
|
return sdkerr
|
|
}
|
|
|
|
func (sdk mfSDK) Assign(actions []string, userID, groupID, token string) errors.SDKError {
|
|
policy := Policy{
|
|
Subject: userID,
|
|
Object: groupID,
|
|
Actions: actions,
|
|
}
|
|
return sdk.CreateUserPolicy(policy, token)
|
|
}
|
|
|
|
func (sdk mfSDK) Unassign(userID, groupID, token string) errors.SDKError {
|
|
policy := Policy{
|
|
Subject: userID,
|
|
Object: groupID,
|
|
}
|
|
|
|
return sdk.DeleteUserPolicy(policy, token)
|
|
}
|
|
|
|
func (sdk mfSDK) Connect(conn Connection, token string) errors.SDKError {
|
|
data, err := json.Marshal(conn)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.thingsURL, connectEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusOK)
|
|
|
|
return sdkerr
|
|
}
|
|
|
|
func (sdk mfSDK) Disconnect(connIDs Connection, token string) errors.SDKError {
|
|
data, err := json.Marshal(connIDs)
|
|
if err != nil {
|
|
return errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s", sdk.thingsURL, disconnectEndpoint)
|
|
|
|
_, _, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusNoContent)
|
|
|
|
return sdkerr
|
|
}
|
|
|
|
func (sdk mfSDK) ConnectThing(thingID, channelID, token string) errors.SDKError {
|
|
policy := Policy{
|
|
Subject: thingID,
|
|
Object: channelID,
|
|
}
|
|
|
|
return sdk.CreateThingPolicy(policy, token)
|
|
}
|
|
|
|
func (sdk mfSDK) DisconnectThing(thingID, channelID, token string) errors.SDKError {
|
|
policy := Policy{
|
|
Subject: thingID,
|
|
Object: channelID,
|
|
}
|
|
|
|
return sdk.DeleteThingPolicy(policy, token)
|
|
}
|
|
|
|
func (sdk mfSDK) AuthorizeThing(accessReq AccessRequest, token string) (bool, string, errors.SDKError) {
|
|
data, err := json.Marshal(accessReq)
|
|
if err != nil {
|
|
return false, "", errors.NewSDKError(err)
|
|
}
|
|
|
|
url := fmt.Sprintf("%s/%s/%s/%s", sdk.thingsURL, channelsEndpoint, accessReq.Object, accessEndpoint)
|
|
|
|
_, body, sdkerr := sdk.processRequest(http.MethodPost, url, token, data, nil, http.StatusOK)
|
|
if sdkerr != nil {
|
|
return false, "", sdkerr
|
|
}
|
|
resp := canAccessRes{}
|
|
if err := json.Unmarshal(body, &resp); err != nil {
|
|
return false, "", errors.NewSDKError(err)
|
|
}
|
|
|
|
return resp.Authorized, resp.ThingID, nil
|
|
}
|