mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
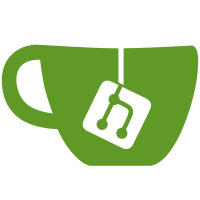
* Replace error messages for things and channels with error messages for entities Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Add db and cache errors Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Migrate swagger to openapi Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Remove db and cache specific err msgs and generalize errs Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Redefine status codes Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Define endpoint error codes in service and swagger Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Fix tests Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Refactor sdk errors Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Fix grpc tests Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com> * Reformat and add err check Signed-off-by: Darko Draskovic <darko.draskovic@gmail.com>
73 lines
1.7 KiB
Go
73 lines
1.7 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package redis
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/go-redis/redis"
|
|
"github.com/mainflux/mainflux/pkg/errors"
|
|
"github.com/mainflux/mainflux/things"
|
|
)
|
|
|
|
const (
|
|
keyPrefix = "thing_key"
|
|
idPrefix = "thing"
|
|
)
|
|
|
|
var _ things.ThingCache = (*thingCache)(nil)
|
|
|
|
type thingCache struct {
|
|
client *redis.Client
|
|
}
|
|
|
|
// NewThingCache returns redis thing cache implementation.
|
|
func NewThingCache(client *redis.Client) things.ThingCache {
|
|
return &thingCache{
|
|
client: client,
|
|
}
|
|
}
|
|
|
|
func (tc *thingCache) Save(_ context.Context, thingKey string, thingID string) error {
|
|
tkey := fmt.Sprintf("%s:%s", keyPrefix, thingKey)
|
|
if err := tc.client.Set(tkey, thingID, 0).Err(); err != nil {
|
|
return errors.Wrap(things.ErrCreateEntity, err)
|
|
}
|
|
|
|
tid := fmt.Sprintf("%s:%s", idPrefix, thingID)
|
|
if err := tc.client.Set(tid, thingKey, 0).Err(); err != nil {
|
|
return errors.Wrap(things.ErrCreateEntity, err)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (tc *thingCache) ID(_ context.Context, thingKey string) (string, error) {
|
|
tkey := fmt.Sprintf("%s:%s", keyPrefix, thingKey)
|
|
thingID, err := tc.client.Get(tkey).Result()
|
|
if err != nil {
|
|
return "", errors.Wrap(things.ErrNotFound, err)
|
|
}
|
|
|
|
return thingID, nil
|
|
}
|
|
|
|
func (tc *thingCache) Remove(_ context.Context, thingID string) error {
|
|
tid := fmt.Sprintf("%s:%s", idPrefix, thingID)
|
|
key, err := tc.client.Get(tid).Result()
|
|
// Redis returns Nil Reply when key does not exist.
|
|
if err == redis.Nil {
|
|
return nil
|
|
}
|
|
if err != nil {
|
|
return errors.Wrap(things.ErrRemoveEntity, err)
|
|
}
|
|
|
|
tkey := fmt.Sprintf("%s:%s", keyPrefix, key)
|
|
if err := tc.client.Del(tkey, tid).Err(); err != nil {
|
|
return errors.Wrap(things.ErrRemoveEntity, err)
|
|
}
|
|
return nil
|
|
}
|