mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
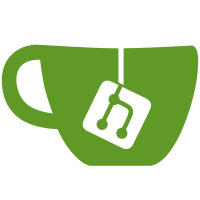
* allow admin to fetch all things Signed-off-by: Burak Sekili <buraksekili@gmail.com> * enable users to fetch their own things via owner field in db Signed-off-by: Burak Sekili <buraksekili@gmail.com> * add listpolicies RPC Signed-off-by: Burak Sekili <buraksekili@gmail.com> * add listPolicies gRPC methods for client and server, and update keto initialization Signed-off-by: Burak Sekili <buraksekili@gmail.com> * update fetching things method Signed-off-by: Burak Sekili <buraksekili@gmail.com> * remove log Signed-off-by: Burak Sekili <buraksekili@gmail.com> * update retrieving policies Signed-off-by: Burak Sekili <buraksekili@gmail.com> * fix linter error Signed-off-by: Burak Sekili <buraksekili@gmail.com> * update mock Signed-off-by: Burak Sekili <buraksekili@gmail.com> * remove checking subject set while parsing subject sets Signed-off-by: Burak Sekili <buraksekili@gmail.com> * move subject declaration to constant value Signed-off-by: Burak Sekili <buraksekili@gmail.com>
73 lines
2.5 KiB
Go
73 lines
2.5 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package auth
|
|
|
|
import (
|
|
"context"
|
|
|
|
acl "github.com/ory/keto/proto/ory/keto/acl/v1alpha1"
|
|
)
|
|
|
|
// PolicyReq represents an argument struct for making a policy related
|
|
// function calls.
|
|
type PolicyReq struct {
|
|
Subject string
|
|
Object string
|
|
Relation string
|
|
}
|
|
|
|
type PolicyPage struct {
|
|
Policies []string
|
|
}
|
|
|
|
// Authz represents a authorization service. It exposes
|
|
// functionalities through `auth` to perform authorization.
|
|
type Authz interface {
|
|
// Authorize checks authorization of the given `subject`. Basically,
|
|
// Authorize verifies that Is `subject` allowed to `relation` on
|
|
// `object`. Authorize returns a non-nil error if the subject has
|
|
// no relation on the object (which simply means the operation is
|
|
// denied).
|
|
Authorize(ctx context.Context, pr PolicyReq) error
|
|
|
|
// AddPolicy creates a policy for the given subject, so that, after
|
|
// AddPolicy, `subject` has a `relation` on `object`. Returns a non-nil
|
|
// error in case of failures.
|
|
AddPolicy(ctx context.Context, pr PolicyReq) error
|
|
|
|
// AddPolicies adds new policies for given subjects. This method is
|
|
// only allowed to use as an admin.
|
|
AddPolicies(ctx context.Context, token, object string, subjectIDs, relations []string) error
|
|
|
|
// DeletePolicy removes a policy.
|
|
DeletePolicy(ctx context.Context, pr PolicyReq) error
|
|
|
|
// DeletePolicies deletes policies for given subjects. This method is
|
|
// only allowed to use as an admin.
|
|
DeletePolicies(ctx context.Context, token, object string, subjectIDs, relations []string) error
|
|
|
|
// ListPolicies lists policies based on the given PolicyReq structure.
|
|
ListPolicies(ctx context.Context, pr PolicyReq) (PolicyPage, error)
|
|
}
|
|
|
|
// PolicyAgent facilitates the communication to authorization
|
|
// services and implements Authz functionalities for certain
|
|
// authorization services (e.g. ORY Keto).
|
|
type PolicyAgent interface {
|
|
// CheckPolicy checks if the subject has a relation on the object.
|
|
// It returns a non-nil error if the subject has no relation on
|
|
// the object (which simply means the operation is denied).
|
|
CheckPolicy(ctx context.Context, pr PolicyReq) error
|
|
|
|
// AddPolicy creates a policy for the given subject, so that, after
|
|
// AddPolicy, `subject` has a `relation` on `object`. Returns a non-nil
|
|
// error in case of failures.
|
|
AddPolicy(ctx context.Context, pr PolicyReq) error
|
|
|
|
// DeletePolicy removes a policy.
|
|
DeletePolicy(ctx context.Context, pr PolicyReq) error
|
|
|
|
RetrievePolicies(ctx context.Context, pr PolicyReq) ([]*acl.RelationTuple, error)
|
|
}
|