mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-24 13:48:49 +08:00
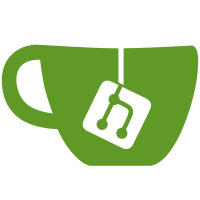
* feat(docker): add trace ration and max conn This adds a new environment variable `MF_JAEGER_TRACE_RATIO` to the `docker/.env` file. The variable is used to set the ratio of requests traced. Additionally, this commit also adds a new environment variable `MF_POSTGRES_MAX_CONNECTIONS` for configuring the maximum number of connections for the Postgres database. These changes are made to enhance the configuration and scalability of the core services. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * Remove comment to trace ratio Signed-off-by: rodneyosodo <blackd0t@protonmail.com> * Reduce postgres max connection to 100 Signed-off-by: rodneyosodo <blackd0t@protonmail.com> --------- Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> Signed-off-by: rodneyosodo <blackd0t@protonmail.com>
62 lines
1.5 KiB
Go
62 lines
1.5 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package jaeger
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
|
|
"go.opentelemetry.io/otel"
|
|
"go.opentelemetry.io/otel/attribute"
|
|
jaegerexp "go.opentelemetry.io/otel/exporters/jaeger"
|
|
"go.opentelemetry.io/otel/sdk/resource"
|
|
tracesdk "go.opentelemetry.io/otel/sdk/trace"
|
|
semconv "go.opentelemetry.io/otel/semconv/v1.12.0"
|
|
)
|
|
|
|
var (
|
|
errNoURL = errors.New("URL is empty")
|
|
errNoSvcName = errors.New("service Name is empty")
|
|
)
|
|
|
|
// NewProvider initializes Jaeger TraceProvider.
|
|
func NewProvider(svcName, url, instanceID string, fraction float64) (*tracesdk.TracerProvider, error) {
|
|
if url == "" {
|
|
return nil, errNoURL
|
|
}
|
|
|
|
if svcName == "" {
|
|
return nil, errNoSvcName
|
|
}
|
|
|
|
exporter, err := jaegerexp.New(jaegerexp.WithCollectorEndpoint(jaegerexp.WithEndpoint(url)))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
attributes := []attribute.KeyValue{
|
|
semconv.ServiceNameKey.String(svcName),
|
|
attribute.String("host.id", instanceID),
|
|
}
|
|
|
|
hostAttr, err := resource.New(context.TODO(), resource.WithHost(), resource.WithOSDescription(), resource.WithContainer())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
attributes = append(attributes, hostAttr.Attributes()...)
|
|
|
|
tp := tracesdk.NewTracerProvider(
|
|
tracesdk.WithSampler(tracesdk.TraceIDRatioBased(fraction)),
|
|
tracesdk.WithBatcher(exporter),
|
|
tracesdk.WithResource(resource.NewWithAttributes(
|
|
semconv.SchemaURL,
|
|
attributes...,
|
|
)),
|
|
)
|
|
otel.SetTracerProvider(tp)
|
|
// otel.SetTextMapPropagator(jaegerp.Jaeger{})
|
|
|
|
return tp, nil
|
|
}
|