mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
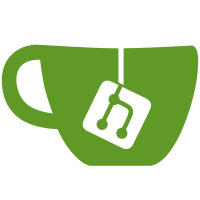
* Change service Default ports Updated ports: - auth http 9000 - auth grpc 7000 - provision http 9001 - things http 9002 - things auth http 9003 - things auth grpc 7001 - twins http 9004 - users http 9005 - bootstrap http 9006 - cassandra-reader http 9007 - cassandra-writer http 9008 - influxdb-reader http 9009 - influxdb-writer http 9010 - lora http 9011 - mongodb reader http 9012 - mongodb writer http 9013 - postgres-reader http 9014 - postgrs-writer http 9015 - smpp-notifier http 9016 - smtp-notifier http 9017 - timescale-reader http 9018 - timescale-writer http 9019 Signed-off-by: SammyOina <sammyoina@gmail.com> * change default service ports on env and sh Signed-off-by: SammyOina <sammyoina@gmail.com> * change things url default port Signed-off-by: SammyOina <sammyoina@gmail.com> * change default ports order by importance - auth http 9000 - auth grpc 7000 - things http 9001 - things auth http 9002 - things auth grpc 7001 - users http 9003 - cassandra-reader http 9004 - cassandra-writer http 9005 - influxdb-reader http 9006 - influxdb-writer http 9007 - mongodb reader http 9008 - mongodb writer http 9009 - postgres-reader http 9010 - postgres-writer http 9011 - timescale-reader http 9012 - timescale-writer http 9013 - bootstrap http 9014 - smpp-notifier http 9015 - smtp-notifier http 9016 - provision http 9017 - lora http 9018 - twins http 9019 Signed-off-by: SammyOina <sammyoina@gmail.com> * lower port number in auth service Signed-off-by: SammyOina <sammyoina@gmail.com> * change things and users port - things 9000 - things auth 9001 - things auth grpc 7000 - users 9002 Signed-off-by: SammyOina <sammyoina@gmail.com> * update documentaton to new port numbers Signed-off-by: SammyOina <sammyoina@gmail.com> * update test and metrics Signed-off-by: SammyOina <sammyoina@gmail.com> * update host on metrics Signed-off-by: SammyOina <sammyoina@gmail.com> * resolving conflics Signed-off-by: SammyOina <sammyoina@gmail.com> * set http adapter port to :80 Signed-off-by: SammyOina <sammyoina@gmail.com> * reassign http port on metrics to :80 Signed-off-by: SammyOina <sammyoina@gmail.com> * reassign http adapter port Signed-off-by: SammyOina <sammyoina@gmail.com> * set http adapter port to 8008 Signed-off-by: SammyOina <sammyoina@gmail.com> * document http adapter default port Signed-off-by: SammyOina <sammyoina@gmail.com> --------- Signed-off-by: SammyOina <sammyoina@gmail.com>
56 lines
1.4 KiB
Go
56 lines
1.4 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package grpc_test
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"os"
|
|
"testing"
|
|
|
|
"github.com/mainflux/mainflux"
|
|
"github.com/mainflux/mainflux/pkg/uuid"
|
|
"github.com/mainflux/mainflux/things"
|
|
grpcapi "github.com/mainflux/mainflux/things/api/auth/grpc"
|
|
"github.com/mainflux/mainflux/things/mocks"
|
|
"github.com/opentracing/opentracing-go/mocktracer"
|
|
"google.golang.org/grpc"
|
|
)
|
|
|
|
const (
|
|
port = 7000
|
|
token = "token"
|
|
wrong = "wrong"
|
|
email = "john.doe@email.com"
|
|
)
|
|
|
|
var svc things.Service
|
|
|
|
func TestMain(m *testing.M) {
|
|
startServer()
|
|
code := m.Run()
|
|
os.Exit(code)
|
|
}
|
|
|
|
func startServer() {
|
|
svc = newService(map[string]string{token: email})
|
|
listener, _ := net.Listen("tcp", fmt.Sprintf(":%d", port))
|
|
server := grpc.NewServer()
|
|
mainflux.RegisterThingsServiceServer(server, grpcapi.NewServer(mocktracer.New(), svc))
|
|
go server.Serve(listener)
|
|
}
|
|
|
|
func newService(tokens map[string]string) things.Service {
|
|
policies := []mocks.MockSubjectSet{{Object: "users", Relation: "member"}}
|
|
auth := mocks.NewAuthService(tokens, map[string][]mocks.MockSubjectSet{email: policies})
|
|
conns := make(chan mocks.Connection)
|
|
thingsRepo := mocks.NewThingRepository(conns)
|
|
channelsRepo := mocks.NewChannelRepository(thingsRepo, conns)
|
|
chanCache := mocks.NewChannelCache()
|
|
thingCache := mocks.NewThingCache()
|
|
idProvider := uuid.NewMock()
|
|
|
|
return things.New(auth, thingsRepo, channelsRepo, chanCache, thingCache, idProvider)
|
|
}
|