mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
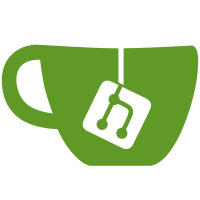
* Add mongodb reader service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add tests for mongodb reader service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Add documentation for mongodb reader service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Fix test function name Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com> * Update comment in docker-compose for mongodb-reader service Signed-off-by: Aleksandar Novakovic <aleksandar.novakovic@mainflux.com>
46 lines
1.1 KiB
Go
46 lines
1.1 KiB
Go
package mongodb
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/mainflux/mainflux"
|
|
"github.com/mainflux/mainflux/readers"
|
|
"github.com/mongodb/mongo-go-driver/bson"
|
|
"github.com/mongodb/mongo-go-driver/mongo"
|
|
"github.com/mongodb/mongo-go-driver/mongo/findopt"
|
|
)
|
|
|
|
const collection = "mainflux"
|
|
|
|
var _ readers.MessageRepository = (*mongoRepository)(nil)
|
|
|
|
type mongoRepository struct {
|
|
db *mongo.Database
|
|
}
|
|
|
|
// New returns new MongoDB reader.
|
|
func New(db *mongo.Database) readers.MessageRepository {
|
|
return mongoRepository{db: db}
|
|
}
|
|
|
|
func (repo mongoRepository) ReadAll(chanID, offset, limit uint64) []mainflux.Message {
|
|
col := repo.db.Collection(collection)
|
|
cursor, err := col.Find(context.Background(), bson.NewDocument(bson.EC.Int64("channel", int64(chanID))), findopt.Limit(int64(limit)), findopt.Skip(int64(offset)))
|
|
if err != nil {
|
|
return []mainflux.Message{}
|
|
}
|
|
defer cursor.Close(context.Background())
|
|
|
|
messages := []mainflux.Message{}
|
|
for cursor.Next(context.Background()) {
|
|
var msg mainflux.Message
|
|
if err := cursor.Decode(&msg); err != nil {
|
|
return []mainflux.Message{}
|
|
}
|
|
|
|
messages = append(messages, msg)
|
|
}
|
|
|
|
return messages
|
|
}
|