mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-26 13:48:53 +08:00
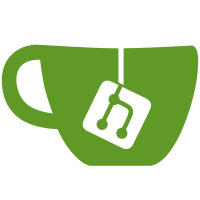
* Update dependencies Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com> * Fix compose files and configs Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com> * Upgrade image versions Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com> * Update Postgres version Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com> * Update test dependencies Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com> * Fix fkey error handling Signed-off-by: dusanb94 <dusan.borovcanin@mainflux.com>
66 lines
1.3 KiB
Go
66 lines
1.3 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package redis
|
|
|
|
import (
|
|
"context"
|
|
"strconv"
|
|
"time"
|
|
|
|
"github.com/go-redis/redis/v8"
|
|
)
|
|
|
|
const (
|
|
streamID = "mainflux.mqtt"
|
|
streamLen = 1000
|
|
)
|
|
|
|
// EventStore is a struct used to store event streams in Redis
|
|
type EventStore struct {
|
|
client *redis.Client
|
|
instance string
|
|
}
|
|
|
|
// NewEventStore returns wrapper around mProxy service that sends
|
|
// events to event store.
|
|
func NewEventStore(client *redis.Client, instance string) EventStore {
|
|
return EventStore{
|
|
client: client,
|
|
instance: instance,
|
|
}
|
|
}
|
|
|
|
func (es EventStore) storeEvent(clientID, eventType string) error {
|
|
timestamp := strconv.FormatInt(time.Now().Unix(), 10)
|
|
|
|
event := mqttEvent{
|
|
clientID: clientID,
|
|
timestamp: timestamp,
|
|
eventType: eventType,
|
|
instance: es.instance,
|
|
}
|
|
|
|
record := &redis.XAddArgs{
|
|
Stream: streamID,
|
|
MaxLenApprox: streamLen,
|
|
Values: event.Encode(),
|
|
}
|
|
|
|
if err := es.client.XAdd(context.Background(), record).Err(); err != nil {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// Connect issues event on MQTT CONNECT
|
|
func (es EventStore) Connect(clientID string) error {
|
|
return es.storeEvent(clientID, "connect")
|
|
}
|
|
|
|
// Disconnect issues event on MQTT CONNECT
|
|
func (es EventStore) Disconnect(clientID string) error {
|
|
return es.storeEvent(clientID, "disconnect")
|
|
}
|