mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
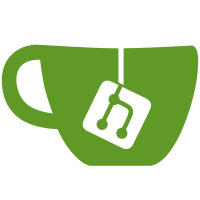
* NOISSUE - Add admin method in users service to return users list Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix loggings and metrics Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add email and metadata filters Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add comment Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Retrieve User infos by ID if Admin Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Remove admin checks and fix comments Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix missing query Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Use generic funccs to create email and metadata queries Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add /users/profile endpoint Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Simplify db helpers Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix View, List, Retrieve prefix methods naming Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix tracer endpoints naming Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix comment Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Fix typo Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com> * Add tests and remove TODO comments Signed-off-by: Manuel Imperiale <manuel.imperiale@gmail.com>
274 lines
9.5 KiB
Go
274 lines
9.5 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package api
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"time"
|
|
|
|
log "github.com/mainflux/mainflux/logger"
|
|
"github.com/mainflux/mainflux/users"
|
|
)
|
|
|
|
var _ users.Service = (*loggingMiddleware)(nil)
|
|
|
|
type loggingMiddleware struct {
|
|
logger log.Logger
|
|
svc users.Service
|
|
}
|
|
|
|
// LoggingMiddleware adds logging facilities to the core service.
|
|
func LoggingMiddleware(svc users.Service, logger log.Logger) users.Service {
|
|
return &loggingMiddleware{logger, svc}
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Register(ctx context.Context, user users.User) (uid string, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method register for user %s took %s to complete", user.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
|
|
}(time.Now())
|
|
|
|
return lm.svc.Register(ctx, user)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Login(ctx context.Context, user users.User) (token string, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method login for user %s took %s to complete", user.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Login(ctx, user)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ViewUser(ctx context.Context, token, id string) (u users.User, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_user for user %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ViewUser(ctx, token, id)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ViewProfile(ctx context.Context, token string) (u users.User, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_profile for usser %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ViewProfile(ctx, token)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListUsers(ctx context.Context, token string, offset, limit uint64, email string, um users.Metadata) (e users.UserPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_users for token %s took %s to complete", token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListUsers(ctx, token, offset, limit, email, um)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) UpdateUser(ctx context.Context, token string, u users.User) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method update_user for user %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.UpdateUser(ctx, token, u)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) GenerateResetToken(ctx context.Context, email, host string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method generate_reset_token for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.GenerateResetToken(ctx, email, host)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ChangePassword(ctx context.Context, email, password, oldPassword string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method change_password for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ChangePassword(ctx, email, password, oldPassword)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ResetPassword(ctx context.Context, email, password string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method reset_password for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ResetPassword(ctx, email, password)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) SendPasswordReset(ctx context.Context, host, email, token string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method send_password_reset for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.SendPasswordReset(ctx, host, email, token)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) CreateGroup(ctx context.Context, token string, group users.Group) (u users.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method create_group with name %s took %s to complete", group.Name, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.CreateGroup(ctx, token, group)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListGroups(ctx context.Context, token, id string, offset, limit uint64, um users.Metadata) (e users.GroupPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_groups for parent %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListGroups(ctx, token, id, offset, limit, um)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListMembers(ctx context.Context, token, id string, offset, limit uint64, um users.Metadata) (e users.UserPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_members for parent %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListMembers(ctx, token, id, offset, limit, um)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) RemoveGroup(ctx context.Context, token, id string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method remove_group with id %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.RemoveGroup(ctx, token, id)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) UpdateGroup(ctx context.Context, token string, group users.Group) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method update_group %s took %s to complete", group.Name, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.UpdateGroup(ctx, token, group)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ViewGroup(ctx context.Context, token, id string) (u users.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_group with id %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ViewGroup(ctx, token, id)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Assign(ctx context.Context, token, userID, groupID string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method assign user %s, group %s took %s to complete", userID, groupID, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Assign(ctx, token, userID, groupID)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Unassign(ctx context.Context, token, userID, groupID string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method unassign for user %s, group %s took %s to complete", userID, groupID, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Unassign(ctx, token, userID, groupID)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListMemberships(ctx context.Context, token, id string, offset, limit uint64, um users.Metadata) (e users.GroupPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_memberships for user %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListMemberships(ctx, token, id, offset, limit, um)
|
|
}
|