mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-27 13:48:49 +08:00
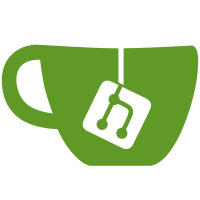
* Initial commit Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * change active to string Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Set default Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Fix query all users Signed-off-by: GitHub <noreply@github.com> * Set user active on service Signed-off-by: GitHub <noreply@github.com> * Rename active to state Signed-off-by: GitHub <noreply@github.com> * check user active on service Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * format Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * format Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * fix test Signed-off-by: GitHub <noreply@github.com> * Add deactivate user tests Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Rename deactivate to change user status Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Revert to sorting users Signed-off-by: GitHub <noreply@github.com> * change user state Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Change user status to enable and disable Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * change user state to status Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * from enable to activate Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * from activate to enable Signed-off-by: GitHub <noreply@github.com> * not found error by retrievebyID Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Combine enable and disable user Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * Add api docs Signed-off-by: b1ackd0t <blackd0t@protonmail.com> * verify docs Signed-off-by: b1ackd0t <blackd0t@protonmail.com> * change to camel Signed-off-by: b1ackd0t <blackd0t@protonmail.com> * Reword Signed-off-by: b1ackd0t <blackd0t@protonmail.com> * fix default state Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * change from VARCHAR to ENUM Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> * invalid user status test Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> Signed-off-by: 0x6f736f646f <blackd0t@protonmail.com> Signed-off-by: GitHub <noreply@github.com> Signed-off-by: b1ackd0t <blackd0t@protonmail.com> Co-authored-by: Dušan Borovčanin <dusan.borovcanin@mainflux.com>
198 lines
6.5 KiB
Go
198 lines
6.5 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
//go:build !test
|
|
|
|
package api
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"time"
|
|
|
|
log "github.com/mainflux/mainflux/logger"
|
|
"github.com/mainflux/mainflux/users"
|
|
)
|
|
|
|
var _ users.Service = (*loggingMiddleware)(nil)
|
|
|
|
type loggingMiddleware struct {
|
|
logger log.Logger
|
|
svc users.Service
|
|
}
|
|
|
|
// LoggingMiddleware adds logging facilities to the core service.
|
|
func LoggingMiddleware(svc users.Service, logger log.Logger) users.Service {
|
|
return &loggingMiddleware{logger, svc}
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Register(ctx context.Context, token string, user users.User) (uid string, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method register for user %s took %s to complete", user.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
|
|
}(time.Now())
|
|
|
|
return lm.svc.Register(ctx, token, user)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Login(ctx context.Context, user users.User) (token string, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method login for user %s took %s to complete", user.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Login(ctx, user)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ViewUser(ctx context.Context, token, id string) (u users.User, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_user for user %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ViewUser(ctx, token, id)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ViewProfile(ctx context.Context, token string) (u users.User, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_profile for user %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ViewProfile(ctx, token)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListUsers(ctx context.Context, token string, pm users.PageMetadata) (e users.UserPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_users for token %s took %s to complete", token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListUsers(ctx, token, pm)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) UpdateUser(ctx context.Context, token string, u users.User) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method update_user for user %s took %s to complete", u.Email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.UpdateUser(ctx, token, u)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) GenerateResetToken(ctx context.Context, email, host string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method generate_reset_token for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.GenerateResetToken(ctx, email, host)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ChangePassword(ctx context.Context, email, password, oldPassword string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method change_password for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ChangePassword(ctx, email, password, oldPassword)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ResetPassword(ctx context.Context, email, password string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method reset_password for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ResetPassword(ctx, email, password)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) SendPasswordReset(ctx context.Context, host, email, token string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method send_password_reset for user %s took %s to complete", email, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.SendPasswordReset(ctx, host, email, token)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) ListMembers(ctx context.Context, token, groupID string, pm users.PageMetadata) (mp users.UserPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_members for group %s took %s to complete", groupID, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.ListMembers(ctx, token, groupID, pm)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) EnableUser(ctx context.Context, token string, id string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method enable_user for user %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.EnableUser(ctx, token, id)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) DisableUser(ctx context.Context, token string, id string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method disable_user for user %s took %s to complete", id, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.DisableUser(ctx, token, id)
|
|
}
|