mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-28 13:48:49 +08:00
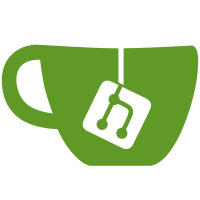
* initial commit Signed-off-by: aryan <aryangodara03@gmail.com> * remove unused variables. Signed-off-by: aryan <aryangodara03@gmail.com> * removed temporarily created file. Signed-off-by: aryan <aryangodara03@gmail.com> * Fix failing CI Signed-off-by: aryan <aryangodara03@gmail.com> * Fix thing_test failing cases. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove dead code, debug statements, and add comments. Signed-off-by: aryan <aryangodara03@gmail.com> * Extract errors to separate file. Signed-off-by: aryan <aryangodara03@gmail.com> * Updated things/api/http tests Signed-off-by: aryan <aryangodara03@gmail.com> * Created custom SDK error. Signed-off-by: aryan <aryangodara03@gmail.com> * Changed to using CheckError. All tests passing. Signed-off-by: aryan <aryangodara03@gmail.com> * Replace error interface with errors.SDKError interface. Signed-off-by: aryan <aryangodara03@gmail.com> * Fix failing CI. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove unused sdk errors. Signed-off-by: aryan <aryangodara03@gmail.com> * Change SDKError to error in internal function of sdk package. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove unused error. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove encodeError. All tests working. Signed-off-by: aryan <aryangodara03@gmail.com> * Rename sdkerr vars, convert common strings to constants. Signed-off-by: aryan <aryangodara03@gmail.com> * Change checkerror to take error instead of string. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove unused errors, and removed errfailedwhitelist wrap. Signed-off-by: aryan <aryangodara03@gmail.com> * Removed unused errors, and remove errors.go since it only had a repeated error from errors package Signed-off-by: aryan <aryangodara03@gmail.com> * Remove unused errors. Signed-off-by: aryan <aryangodara03@gmail.com> * Update sdk_error. Signed-off-by: aryan <aryangodara03@gmail.com> * Used function to reduce code for sending and receiving requests. Signed-off-by: aryan <aryangodara03@gmail.com> * Added function sendrequestandgetheadersorerror. Signed-off-by: aryan <aryangodara03@gmail.com> * sdk_error updated. Signed-off-by: aryan <aryangodara03@gmail.com> * Updated function names to processRequest. Signed-off-by: aryan <aryangodara03@gmail.com> * Made errors internal, fixed typo in http. Signed-off-by: aryan <aryangodara03@gmail.com> * Remove empty line. Signed-off-by: aryan <aryangodara03@gmail.com> * merged proceessBody and processHeaders functions in sdk. Signed-off-by: aryan <aryangodara03@gmail.com> * remove sendThingRequest function. Signed-off-by: aryan <aryangodara03@gmail.com> * changed processRequest signature Signed-off-by: aryan <aryangodara03@gmail.com> * changed processRequest signature, changed error names. Signed-off-by: aryan <aryangodara03@gmail.com> Signed-off-by: aryan <aryangodara03@gmail.com> Co-authored-by: Dušan Borovčanin <dusan.borovcanin@mainflux.com>
96 lines
2.2 KiB
Go
96 lines
2.2 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package errors
|
|
|
|
import (
|
|
"encoding/json"
|
|
"errors"
|
|
"fmt"
|
|
"net/http"
|
|
)
|
|
|
|
const err = "error"
|
|
|
|
var (
|
|
// ErrJSONErrKey indicates response body did not contain erorr message.
|
|
errJSONKey = New("response body expected error message json key not found")
|
|
|
|
// ErrUnknown indicates that an unknown error was found in the response body.
|
|
errUnknown = New("unknown error")
|
|
)
|
|
|
|
// SDKError is an error type for Mainflux SDK.
|
|
type SDKError interface {
|
|
Error
|
|
StatusCode() int
|
|
}
|
|
|
|
var _ SDKError = (*sdkError)(nil)
|
|
|
|
type sdkError struct {
|
|
*customError
|
|
statusCode int
|
|
}
|
|
|
|
func (ce *sdkError) Error() string {
|
|
if ce == nil {
|
|
return ""
|
|
}
|
|
if ce.customError == nil {
|
|
return http.StatusText(ce.statusCode)
|
|
}
|
|
return fmt.Sprintf("Status: %s: %s", http.StatusText(ce.statusCode), ce.customError.Error())
|
|
}
|
|
|
|
func (ce *sdkError) StatusCode() int {
|
|
return ce.statusCode
|
|
}
|
|
|
|
// NewSDKError returns an SDK Error that formats as the given text.
|
|
func NewSDKError(err error) SDKError {
|
|
return &sdkError{
|
|
customError: &customError{
|
|
msg: err.Error(),
|
|
err: nil,
|
|
},
|
|
statusCode: 0,
|
|
}
|
|
}
|
|
|
|
// NewSDKErrorWithStatus returns an SDK Error setting the status code.
|
|
func NewSDKErrorWithStatus(err error, statusCode int) SDKError {
|
|
return &sdkError{
|
|
statusCode: statusCode,
|
|
customError: &customError{
|
|
msg: err.Error(),
|
|
err: nil,
|
|
},
|
|
}
|
|
}
|
|
|
|
// CheckError will check the HTTP response status code and matches it with the given status codes.
|
|
// Since multiple status codes can be valid, we can pass multiple status codes to the function.
|
|
// The function then checks for errors in the HTTP response.
|
|
func CheckError(resp *http.Response, expectedStatusCodes ...int) SDKError {
|
|
for _, expectedStatusCode := range expectedStatusCodes {
|
|
if resp.StatusCode == expectedStatusCode {
|
|
return nil
|
|
}
|
|
}
|
|
|
|
var content map[string]interface{}
|
|
if err := json.NewDecoder(resp.Body).Decode(&content); err != nil {
|
|
return NewSDKErrorWithStatus(err, resp.StatusCode)
|
|
}
|
|
|
|
if msg, ok := content[err]; ok {
|
|
if v, ok := msg.(string); ok {
|
|
return NewSDKErrorWithStatus(errors.New(v), resp.StatusCode)
|
|
}
|
|
return NewSDKErrorWithStatus(errUnknown, resp.StatusCode)
|
|
}
|
|
|
|
return NewSDKErrorWithStatus(errJSONKey, resp.StatusCode)
|
|
}
|