mirror of
https://github.com/mainflux/mainflux.git
synced 2025-04-26 13:48:53 +08:00
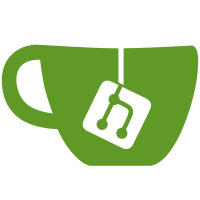
* Fix linting errors Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(linters): add ineffassign linter This commit adds the `ineffassign` linter to the project's `.golangci.yml` configuration file. The `ineffassign` linter helps identify and flag assignments to variables that are never used, helping to improve code quality and maintainability. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * Add extra linters Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(golangci): Add header check - Added goheader check to ensure all files have license headers - Added build tags for "nats" in the .golangci.yml file to include the necessary dependencies for the "nats" package during the build process. - Also, increased the maximum number of issues per linter and the maximum number of same issues reported by the linter to improve the code quality analysis. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> * feat(.golangci.yml): Add new linters Add the following new linters to the .golangci.yml configuration file: - asasalint - asciicheck - bidichk - contextcheck - decorder - dogsled - errchkjson - errname - execinquery - exportloopref - ginkgolinter - gocheckcompilerdirectives These linters will help improve code quality and catch potential issues during the code review process. Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com> --------- Signed-off-by: Rodney Osodo <28790446+rodneyosodo@users.noreply.github.com>
150 lines
6.4 KiB
Go
150 lines
6.4 KiB
Go
// Copyright (c) Mainflux
|
|
// SPDX-License-Identifier: Apache-2.0
|
|
|
|
package api
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"time"
|
|
|
|
mflog "github.com/mainflux/mainflux/logger"
|
|
"github.com/mainflux/mainflux/pkg/groups"
|
|
)
|
|
|
|
var _ groups.Service = (*loggingMiddleware)(nil)
|
|
|
|
type loggingMiddleware struct {
|
|
logger mflog.Logger
|
|
svc groups.Service
|
|
}
|
|
|
|
// LoggingMiddleware adds logging facilities to the groups service.
|
|
func LoggingMiddleware(svc groups.Service, logger mflog.Logger) groups.Service {
|
|
return &loggingMiddleware{logger, svc}
|
|
}
|
|
|
|
// CreateGroup logs the create_group request. It logs the group name, id and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) CreateGroup(ctx context.Context, token string, group groups.Group) (g groups.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method create_group for group %s with id %s using token %s took %s to complete", g.Name, g.ID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.CreateGroup(ctx, token, group)
|
|
}
|
|
|
|
// UpdateGroup logs the update_group request. It logs the group name, id and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) UpdateGroup(ctx context.Context, token string, group groups.Group) (g groups.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method update_group for group %s with id %s using token %s took %s to complete", g.Name, g.ID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.UpdateGroup(ctx, token, group)
|
|
}
|
|
|
|
// ViewGroup logs the view_group request. It logs the group name, id and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) ViewGroup(ctx context.Context, token, id string) (g groups.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method view_group for group %s with id %s using token %s took %s to complete", g.Name, g.ID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.ViewGroup(ctx, token, id)
|
|
}
|
|
|
|
// ListGroups logs the list_groups request. It logs the token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) ListGroups(ctx context.Context, token string, memberKind, memberID string, gp groups.Page) (cg groups.Page, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_groups %d groups using token %s took %s to complete", cg.Total, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.ListGroups(ctx, token, memberKind, memberID, gp)
|
|
}
|
|
|
|
// EnableGroup logs the enable_group request. It logs the group name, id and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) EnableGroup(ctx context.Context, token, id string) (g groups.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method enable_group for group with id %s using token %s took %s to complete", g.ID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.EnableGroup(ctx, token, id)
|
|
}
|
|
|
|
// DisableGroup logs the disable_group request. It logs the group name, id and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) DisableGroup(ctx context.Context, token, id string) (g groups.Group, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method disable_group for group with id %s using token %s took %s to complete", g.ID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.DisableGroup(ctx, token, id)
|
|
}
|
|
|
|
// ListMembers logs the list_members request. It logs the groupID and token and the time it took to complete the request.
|
|
// If the request fails, it logs the error.
|
|
func (lm *loggingMiddleware) ListMembers(ctx context.Context, token, groupID, permission, memberKind string) (mp groups.MembersPage, err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method list_memberships for group with id %s using token %s took %s to complete", groupID, token, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
return lm.svc.ListMembers(ctx, token, groupID, permission, memberKind)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Assign(ctx context.Context, token, groupID string, relation string, memberKind string, memberIDs ...string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method assign for token %s and member %s group id %s took %s to complete", token, memberIDs, groupID, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Assign(ctx, token, groupID, relation, memberKind, memberIDs...)
|
|
}
|
|
|
|
func (lm *loggingMiddleware) Unassign(ctx context.Context, token string, groupID string, relation string, memberKind string, memberIDs ...string) (err error) {
|
|
defer func(begin time.Time) {
|
|
message := fmt.Sprintf("Method unassign for token %s and member %s group id %s took %s to complete", token, memberIDs, groupID, time.Since(begin))
|
|
if err != nil {
|
|
lm.logger.Warn(fmt.Sprintf("%s with error: %s.", message, err))
|
|
return
|
|
}
|
|
lm.logger.Info(fmt.Sprintf("%s without errors.", message))
|
|
}(time.Now())
|
|
|
|
return lm.svc.Unassign(ctx, token, groupID, relation, memberKind, memberIDs...)
|
|
}
|