# Jade.go - template engine for Go (golang) Package jade (github.com/Joker/jade) is a simple and fast template engine implementing Jade/Pug template. Jade precompiles templates to Go code or generates html/template. Now Jade-lang is renamed to [Pug template engine](https://pugjs.org/language/tags.html). [](https://pkg.go.dev/github.com/Joker/jade#section-documentation) [](https://goreportcard.com/report/github.com/Joker/jade) ## Jade/Pug syntax example: ```jade //- :go:func Index(pageTitle string, youAreUsingJade bool) mixin for(golang) #cmd Precompile jade templates to #{golang} code. doctype html html(lang="en") head title= pageTitle script(type='text/javascript'). if(question){ answer(40 + 2) } body h1 Jade - template engine +for('Go') #container.col if youAreUsingJade p You are amazing else p Get on it! p. Jade/Pug is a terse and simple templating language with a #[strong focus] on performance and powerful features. ``` becomes ```html
You are amazing
Jade/Pug is a terse and simple templating language with a focus on performance and powerful features.
Hello ` hello__1 = `!
` ) func Jade_hello(word string, wr io.Writer) { buffer := &WriterAsBuffer{wr} buffer.WriteString(hello__0) WriteEscString(word, buffer) buffer.WriteString(hello__1) } ``` `main.go` ```go package main //go:generate jade -pkg=main -writer hello.jade import "net/http" func main() { http.HandleFunc("/", func(wr http.ResponseWriter, req *http.Request) { Jade_hello("jade", wr) }) http.ListenAndServe(":8080", nil) } ``` output at localhost:8080 ```htmlHello jade!
``` ### github.com/Joker/jade package generate [`html/template`](https://pkg.go.dev/html/template#hdr-Introduction) at runtime (This case is slightly slower and doesn't support[^2] all features of Jade.go) ```go package main import ( "fmt" "html/template" "net/http" "github.com/Joker/hpp" // Prettify HTML "github.com/Joker/jade" ) func handler(w http.ResponseWriter, r *http.Request) { jadeTpl, _ := jade.Parse("jade", []byte("doctype 5\n html: body: p Hello #{.Word} !")) goTpl, _ := template.New("html").Parse(jadeTpl) fmt.Printf("output:%s\n\n", hpp.PrPrint(jadeTpl)) goTpl.Execute(w, struct{ Word string }{"jade"}) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } ``` console output ```htmlHello {{.Word}} !
``` output at localhost:8080 ```htmlHello jade !
``` ## Performance The data of chart comes from [SlinSo/goTemplateBenchmark](https://github.com/SlinSo/goTemplateBenchmark). 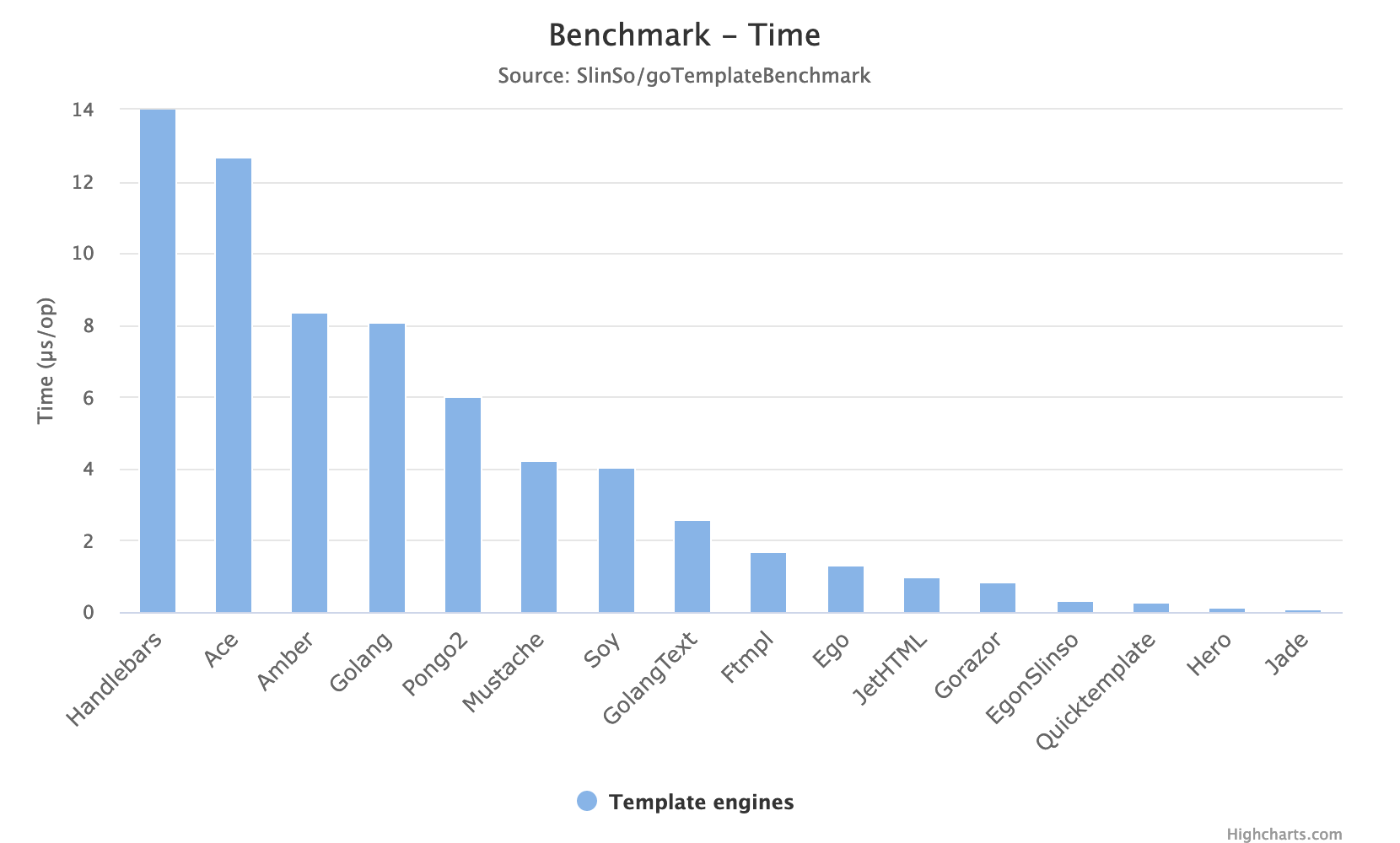 ## Custom filter :go This filter is used as helper for command line tool (to set imports, function name and parameters). Filter may be placed at any nesting level. When Jade used as library :go filter is not needed. ### Nested filter :func ``` :go:func CustomNameForTemplateFunc(any []int, input string, args map[string]int) :go:func(name) OnlyCustomNameForTemplateFunc :go:func(args) (only string, input float32, args uint) ``` ### Nested filter :import ``` :go:import "github.com/Joker/jade" github.com/Joker/hpp ``` #### note [^1]: `Usage: ./jade [OPTION]... [FILE]...` ``` -basedir string base directory for templates (default "./") -d string directory for generated .go files (default "./") -fmt HTML pretty print output for generated functions -inline inline HTML in generated functions -pkg string package name for generated files (default "jade") -stdbuf use bytes.Buffer [default bytebufferpool.ByteBuffer] -stdlib use stdlib functions -writer use io.Writer for output ``` [^2]: Runtime `html/template` generation doesn't support the following features: `=>` means it generate the folowing template ``` for => "{{/* %s, %s */}}{{ range %s }}" for if => "{{ if gt len %s 0 }}{{/* %s, %s */}}{{ range %s }}" multiline code => "{{/* %s */}}" inheritance block => "{{/* block */}}" case statement => "{{/* switch %s */}}" when => "{{/* case %s: */}}" default => "{{/* default: */}}" ``` You can change this behaviour in [`config.go`](https://github.com/Joker/jade/blob/master/config.go#L24) file. Partly this problem can be solved by [custom](https://pkg.go.dev/text/template#example-Template-Func) functions.